Building an iOS App with Go for CRUD Operations
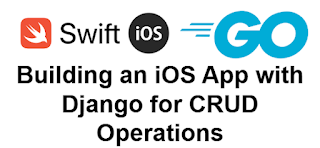
Here’s a complete end-to-end example of a CRUD app using Go for the backend and Swift for the iOS frontend . The app will manage a simple list of tasks. 1. Backend: Go Setup Install Go: https://go.dev/ Install dependencies: Use the Gin framework for HTTP and GORM for database interactions. Install via: go get - u github. com /gin-gonic/gin go get - u gorm.io/gorm go get - u gorm.io/driver/sqlite Code: main.go Here’s the complete code for a simple CRUD API: package main import ( "net/http" "github.com/gin-gonic/gin" "gorm.io/driver/sqlite" "gorm.io/gorm" ) type Task struct { ID uint `json: "id" gorm: "primaryKey" ` Title string `json: "title" ` Description string `json: "description" ` } var db *gorm. DB func main () { // Initialize database var err error db, err = gorm. Open (sqlite. Open ( "tasks.db" ), &gorm. Config {}) ...