Spring Boot MongoDB - Integration Testing with Testcontainers
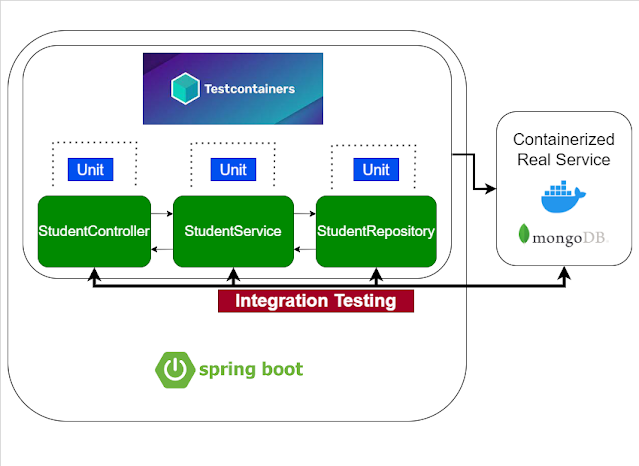
In this section, we will learn how to test Spring Boot, Spring Data MongoDB, and MongoDB based application using Testcontainers and @SpringBootTest. 1. What we will build? We will create a web application with Spring Boot, Spring Data MongoDB and MongoDB. The application will consist of three layer, that is a Controller, a Service, and a Repository layer. Controller layer takes care of mapping request data to the defined request handler method. Once response body is generated from the handler method, it converts it to JSON response. Service layer facilitates communication between the controller and the repository layer. We are using Spring Data MongoDB for managing database operations, so we can use Spring Data MongoRepository interface. **Finally we will do a integration testing with help of Testcontainers to verify our system is working as expected. 2. Testcontainers Testcontainers is an open source testing library that allows us to run docker containers directly in our spring bo