Build a RESTful CRUD API with Laravel and AWS DynamoDB: A Step-by-Step Guide
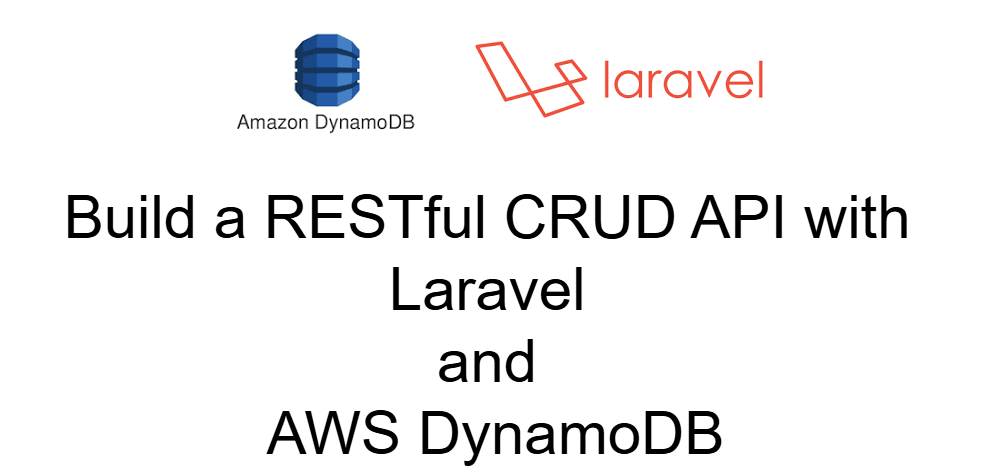
To create a RESTful CRUD API with Laravel and AWS DynamoDB, you need to integrate DynamoDB into your Laravel application and implement basic CRUD (Create, Read, Update, Delete) functionality. Here's a general approach to set this up: Prerequisites Laravel Application : Ensure you have a Laravel application installed. AWS Account : You need an AWS account with DynamoDB access. Composer AWS SDK for PHP : You need the AWS SDK for PHP, which Laravel can use. Step-by-Step Guide 1. Install the AWS SDK for PHP Run the following command to install the AWS SDK for PHP in your Laravel project: composer require aws/aws-sdk-php 2. Set up AWS Configuration You need to configure your AWS credentials. You can either use environment variables or the config/services.php file in Laravel. In .env , add your AWS credentials: AWS_ACCESS_KEY_ID =your-access-key AWS_SECRET_ACCESS_KEY =your-secret-key AWS_DEFAULT_REGION =your-region AWS_DYNAMODB_TABLE =your-table-name In config/services.php , add the fo...