Essential AWS Services Every PHP Developer Should Master!
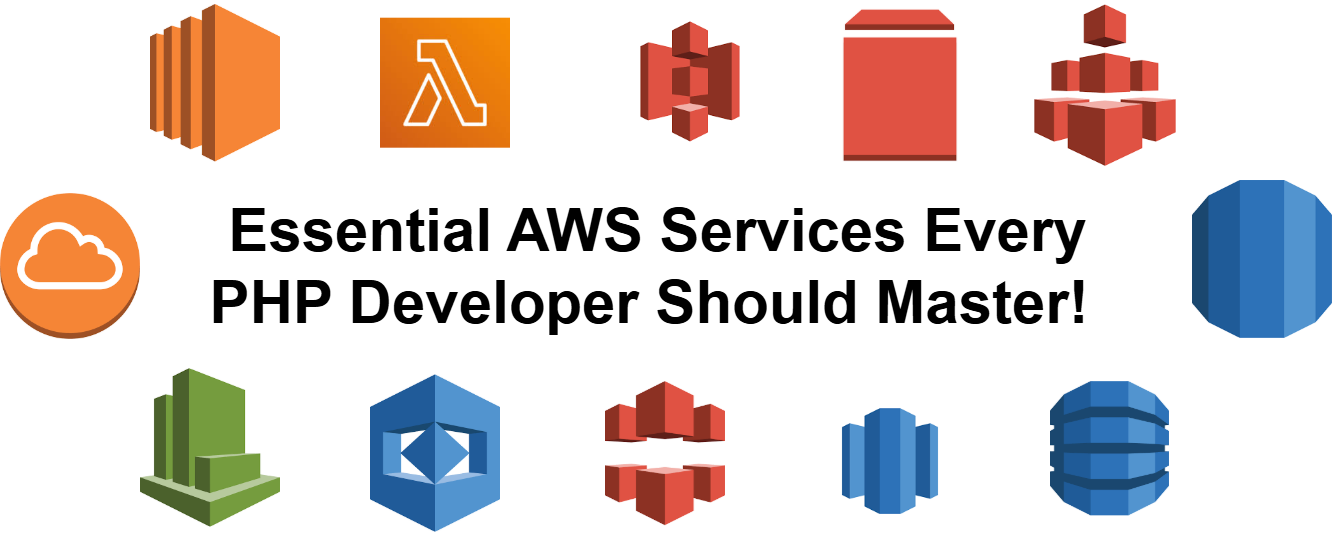
As a PHP developer, mastering AWS services can significantly enhance your ability to scale applications, manage resources, and improve performance. Here's a list of essential AWS services you should familiarize yourself with: 1. Amazon EC2 (Elastic Compute Cloud) 🚀 What it is : EC2 provides resizable compute capacity in the cloud. You can run PHP applications on EC2 instances, allowing you to scale your backend services. Why it's important : It gives you the flexibility to run web applications with different configurations based on traffic and workload. Tip : Use Amazon EC2 Auto Scaling to automatically scale your PHP app based on demand. 2. Amazon S3 (Simple Storage Service) 🗂️ What it is : S3 is an object storage service that allows you to store and retrieve any amount of data at any time. Why it's important : Ideal for storing static assets like images, videos, and backups for your PHP applications. Tip : Use S3 Versioning to track changes to your files and easily ...